High Performance
Xamarin compiles your app to a native binary, not cross-compiled, and not interpreted. Native compilation gives users brilliant app performance for even the most demanding scenarios, like high frame rate gaming and complex data visualizations. With a small footprint (2.5 MB added to your application code), and negligible impact to app startup time, you can build apps that run faster, wherever they run. Full access to hardware acceleration like the GPU.
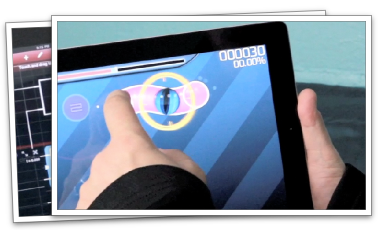