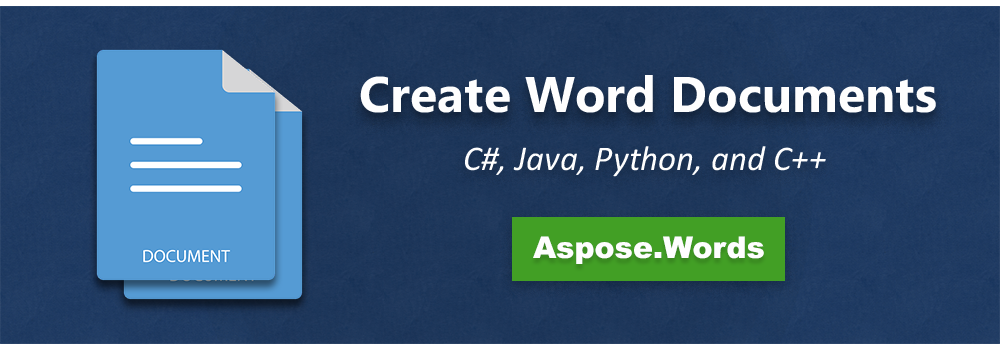
多くのアプリケーションにとってプログラム内で Word 文書を生成、操作することは、一般的な機能要件のひとつです。幸運なことに、このタスクをさまざまなプログラミング言語で簡素化できる強力なライブラリが存在します。その中のひとつに挙げられる多目的なソリューションの Aspose.Words は、その堅牢な API により、Word ファイルをシームレスに生成、編集、変換できます。
この記事では、Aspose.Words を使用して C#、Java、Python、C++ で Word 文書を簡単に生成する方法を紹介します。
- Word 文書を生成できるライブラリ
- C# で Word 文書を生成しましょう
- Java で Word 文書を生成しましょう
- Python で Word 文書を生成しましょう
- C++ で Word 文書を生成しましょう
Word 文書を生成できるライブラリ
Aspose.Words は、Microsoft Word 文書をプログラム内で操作できる人気の API です。コンテンツの生成、変更、変換、抽出など、Word 文書に関連した幅広いタスクを実行できます。Aspose.Words の主な機能には、レポート生成、差し込み印刷、ドキュメント形式の変換、メタデータの操作、およびテンプレートを使用したドキュメント生成なども含まれます。
それでは、さまざまなプログラミング言語で Word 文書を生成する方法について、詳しく見ていきましょう。
C# で Word 文書を生成しましょう
Aspose.Words for .NET は、.NET アプリケーション内で Word 文書を生成、変更、変換、レンダリングできる、ドキュメント処理 API です。Microsoft Word ファイルを操作する包括的な機能が提供されています。C# で Word 文書を生成する手順は次のとおりです。
- 最初に、新規の Word 文書用に Document クラスのインスタンスを生成します。
- DocumentBuilder クラスのインスタンスを生成し、 それを Document オブジェクトで初期化します。
- DocumentBuilder オブジェクトで、テキスト (またはその他の要素) を Word 文書に挿入します。
- 最後に、Document.Save メソッドで Word 文書を保存します。
下記は、C# で DOCX ファイルを生成するサンプル コードです。
// Create a new document
Document doc = new Document();
DocumentBuilder builder = new DocumentBuilder(doc);
// Specify font formatting
Font font = builder.Font;
font.Size = 32;
font.Bold = true;
font.Color = System.Drawing.Color.Black;
font.Name = "Arial";
font.Underline = Underline.Single;
// Insert text
builder.Writeln("This is the first page.");
builder.Writeln();
// Change formatting for next elements.
font.Underline = Underline.None;
font.Size = 10;
font.Color = System.Drawing.Color.Blue;
builder.Writeln("This following is a table");
// Insert a table
Table table = builder.StartTable();
// Insert a cell
builder.InsertCell();
// Use fixed column widths.
table.AutoFit(AutoFitBehavior.AutoFitToContents);
builder.CellFormat.VerticalAlignment = CellVerticalAlignment.Center;
builder.Write("This is row 1 cell 1");
// Insert a cell
builder.InsertCell();
builder.Write("This is row 1 cell 2");
builder.EndRow();
builder.InsertCell();
builder.Write("This is row 2 cell 1");
builder.InsertCell();
builder.Write("This is row 2 cell 2");
builder.EndRow();
builder.EndTable();
builder.Writeln();
// Insert image
builder.InsertImage("image.png");
// Insert page break
builder.InsertBreak(BreakType.PageBreak);
// all the elements after page break will be inserted to next page.
// Save the document
doc.Save("Document.docx");
create-word-file.cs hosted with ❤ by GitHub
Java で Word 文書を生成しましょう
.NET版と同じように、Aspose.Words for Java は、Microsoft Word 文書を Java で操作できる人気の Word 処理ライブラリです。Microsoft Word を使わずに、Word 文書を生成、変更、変換、操作できます。このライブラリで提供される基本的な機能から高度な機能まで、複雑なコードを記述せずにリッチな Word 文書を生成できます。Java で Word 文書を生成する手順は次のとおりです。
- 最初に、Document クラスをインスタンス化します。
- 次に、DocumentBuilder クラスのオブジェクトを生成し、 Document オブジェクトで初期化します。
- テキストを追加する場合は、Font クラスでフォント サイズ、ファミリなどを設定します。
- ParagraphFormat クラスで段落関連のプロパティを設定します。
- DocumentBuilder.write() メソッドで Word 文書にテキストを挿入します。
- 最後に、Document.save() メソッドで Word 文書を保存します。
下記は、Java で DOCX ファイルを生成するサンプル コードです。
// Create a Document object
Document doc = new Document();
// Create a DocumentBuilder object
DocumentBuilder builder = new DocumentBuilder(doc);
// Specify font formatting
Font font = builder.getFont();
font.setSize(18);
font.setBold(true);
font.setColor(Color.BLACK);
font.setName("Arial");
builder.write("How to Create a Rich Word Document?");
builder.insertBreak(BreakType.LINE_BREAK);
// Start the paragraph
font.setSize(12);
font.setBold(false);
ParagraphFormat paragraphFormat = builder.getParagraphFormat();
paragraphFormat.setFirstLineIndent(12);
paragraphFormat.setKeepTogether(true);
builder.write("This article shows how to create a Word document containing text, images and lists.");
// Save the document
doc.save("Rich Word Document.docx");
create-word-file.java hosted with ❤ by GitHub
Python で Word 文書を生成しましょう
Python 環境向けには、Aspose.Words for Python が提供されています。この堅牢で使用方法も簡単なドキュメント処理ライブラリによって、Python アプリケーション内で Word 文書を生成、変更、変換、レンダリングできます。他の Aspose.Words ファミリ製品と同じように、Aspose.Words for Python は、Word 文書を処理できる完全なパッケージです。Python で Word 文書を生成する手順は次のとおりです。
- 最初に、Document クラスのオブジェクトを生成します。
- 次に、DocumentBuilder クラスのオブジェクトを生成します。
- DocumentBuilder.write() メソッドで Word 文書にテキストを挿入します。
- 最後に、Document.save() メソッドを使用して Word 文書を保存します。
下記は、Python で Word 文書を生成するサンプル コードです。
import aspose.words as aw
# create document object
doc = aw.Document()
# create a document builder object
builder = aw.DocumentBuilder(doc)
# add text to the document
builder.write("Hello world!")
# save document
doc.save("out.docx")
create-word-file.py hosted with ❤ by GitHub
C++ で Word 文書を生成しましょう
C++ のアプリケーションにドキュメント処理機能を組み込む必要がある場合は、Aspose.Words for C++ を使用します。C++ アプリケーション内で Word 文書を生成、操作できる強力なライブラリです。さまざまな Word 文書の処理を簡単に実行できます。次の手順で、新規の Word 文書を C++ で簡単に生成できます。
- 最初に、新規の Word 文書を生成するために Document クラスをインスタンス化します。
- 次に、Word 文書にテキストやその他の要素を挿入するための DocumentBuilder クラスのオブジェクトを生成します。
- DocumentBuilder->Writeln() メソッドでテキストを追加します。
- 最後に、Document->Save() メソッドで、ドキュメントを Word 文書として保存します。
下記は、C++ で DOCX を生成するサンプル コードです。
// Initialize a Document
System::SharedPtr<Document> doc = System::MakeObject<Document>();
// Use a document builder to add content to the document
System::SharedPtr<DocumentBuilder> builder = System::MakeObject<DocumentBuilder>(doc);
// Add text
builder->Writeln(u"Hello World!");
// Save the document to disk
doc->Save(u"document.docx");
create-word-file.cpp hosted with ❤ by GitHub
このブログに掲載している例では、さまざまなプログラミング言語における Aspose.Words の基本的な使用方法を紹介しています。どの言語を使用する場合でも、ドキュメントを生成、DocumentBuilder を使ってコンテンツを追加、最後にそのドキュメントを保存、と処理手順は同じです。Aspose.Words では、書式、スタイル、表、画像などを操作できるさまざまな機能が提供される注目の製品です。
C#、Java、Python、C++ など、それらのすべてのプログラミング言語で Word ファイルを生成したり変更したりする際に、Aspose.Words は信頼性の高い選択肢になります。異なる言語間で一貫して設計された API は、開発プロセスを簡素化し、効率的なドキュメント処理を達成できることを保証します。
Aspose 製品では、無償体験版のダウンロードが用意されています。
機能制限のない 30 日間無償の評価ライセンスもありますので、お気軽にお問い合わせください。
以上です。
© Aspose Pty Ltd 2001-2024.
「Create Word Documents in C#, Java, Python, and C++」